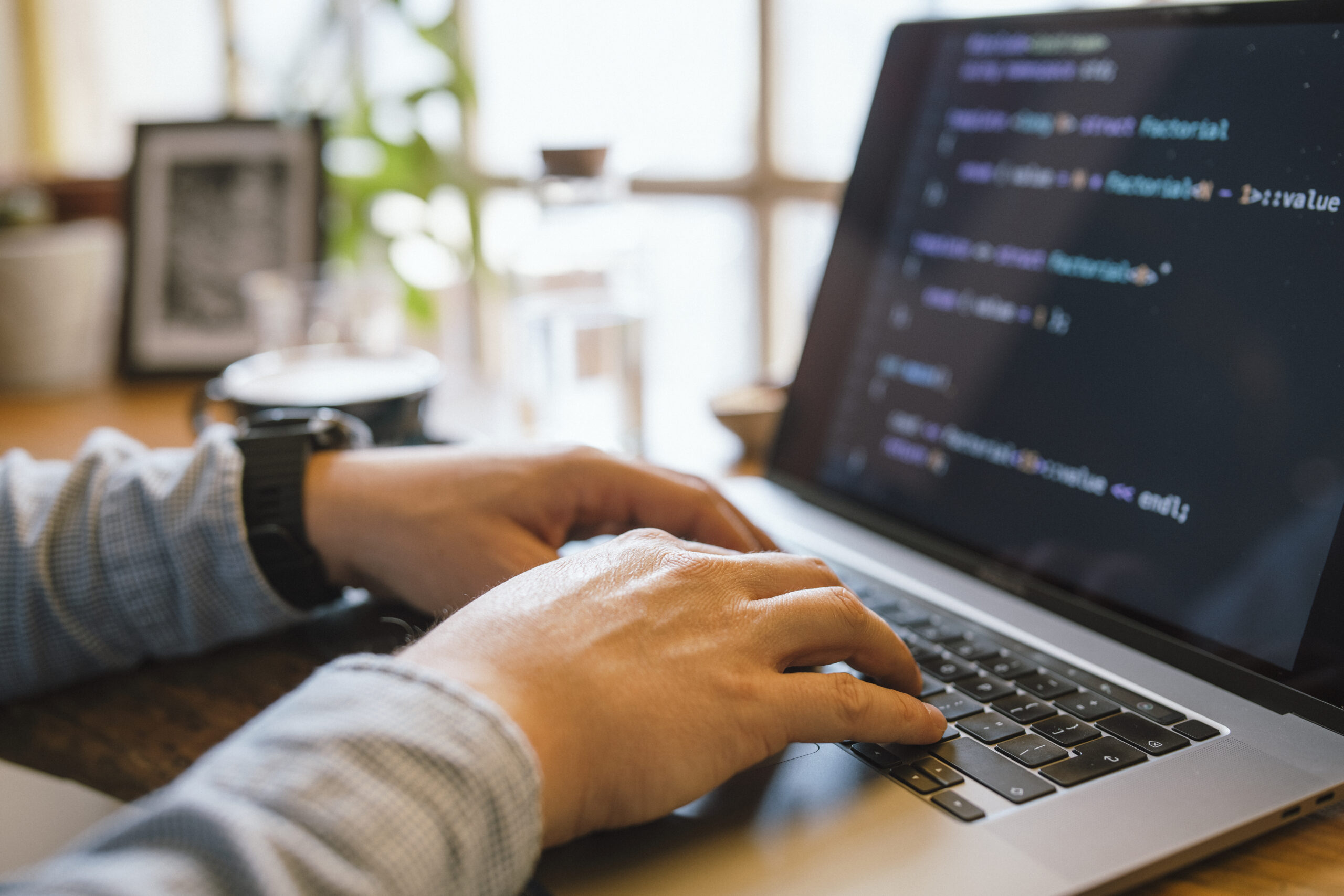
Debugging is The most essential — but typically forgotten — skills inside a developer’s toolkit. It is not almost repairing broken code; it’s about comprehension how and why points go wrong, and learning to Consider methodically to resolve challenges competently. Whether you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can save hours of frustration and significantly enhance your productiveness. Listed below are many approaches to aid developers level up their debugging game by me, Gustavo Woltmann.
Master Your Applications
Among the list of fastest methods developers can elevate their debugging abilities is by mastering the equipment they use every single day. Even though creating code is one Element of progress, figuring out the best way to interact with it correctly for the duration of execution is equally vital. Modern-day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these resources can perform.
Get, for instance, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in some cases modify code around the fly. When made use of accurately, they Allow you to notice precisely how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time effectiveness metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change frustrating UI concerns into workable tasks.
For backend or technique-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Manage about running processes and memory administration. Discovering these resources could have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Edition Management units like Git to know code historical past, find the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates going past default settings and shortcuts — it’s about building an intimate understanding of your growth setting making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you could spend solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Before leaping to the code or producing guesses, developers have to have to produce a consistent environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of likelihood, frequently leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps led to The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the exact problems under which the bug happens.
When you’ve gathered sufficient facts, attempt to recreate the condition in your local atmosphere. This might imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated checks that replicate the edge circumstances or point out transitions involved. These exams don't just assist expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue can be environment-certain — it would materialize only on specific running units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t just a phase — it’s a frame of mind. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at probable fixes properly, and connect extra clearly along with your group or consumers. It turns an abstract complaint into a concrete challenge — Which’s where builders prosper.
Browse and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders need to understand to deal with error messages as immediate communications with the technique. They typically let you know precisely what happened, where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in whole. Several builders, particularly when below time tension, look at the very first line and immediately get started generating assumptions. But deeper from the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — examine and realize them first.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are imprecise or generic, As well as in These situations, it’s very important to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, encouraging you understand what’s happening under the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Deadly. Use DEBUG for comprehensive diagnostic info during development, Facts for normal gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, condition adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specially valuable in creation environments exactly where stepping by code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To effectively determine and correct bugs, builders ought to approach the process like a detective fixing a secret. This mindset allows break down complicated troubles into workable sections and abide by clues logically to uncover the root result in.
Start off by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or effectiveness concerns. The same as a detective surveys against the law scene, collect as much related details as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and person experiences to piece with each other a clear image of what’s taking place.
Up coming, kind hypotheses. Question on your own: What may very well be producing this behavior? Have any variations recently been made towards the codebase? Has this problem occurred before less than very similar situation? The target is usually to slim down choices and determine possible culprits.
Then, test your theories systematically. Attempt to recreate the condition inside of a managed natural environment. For those who suspect a certain operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code issues and Allow the effects guide you closer to the reality.
Shell out close notice to modest specifics. Bugs often cover inside the the very least predicted spots—like a lacking semicolon, an off-by-one particular error, or maybe a race problem. Be complete and individual, resisting the urge to patch the issue with no totally being familiar with it. Short term fixes may cover the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and learned. Just as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and support others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Write Exams
Composing checks is among the most effective methods to increase your debugging skills and General advancement effectiveness. Assessments not simply assistance catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning changes to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These little, isolated tests can quickly expose whether a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know in which to search, drastically lowering time spent debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear following previously staying fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other effortlessly. They’re notably beneficial for catching bugs that occur in advanced techniques with multiple parts or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of understanding The natural way qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is fixed. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—assisting you catch far more bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, get more info it’s easy to become immersed in the trouble—observing your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you're as well close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less effective at issue-solving. A brief stroll, a coffee break, or simply switching to another undertaking for ten–15 minutes can refresh your focus. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do some thing unrelated to code. It may well really feel counterintuitive, In particular below limited deadlines, however it essentially leads to speedier and more effective debugging Eventually.
In brief, getting breaks isn't a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to mirror and examine what went Mistaken.
Get started by inquiring yourself a couple of crucial queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code assessments, or logging? The responses normally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or A fast understanding-sharing session, encouraging Some others stay away from the same challenge boosts group efficiency and cultivates a much better Understanding culture.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders are not those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.